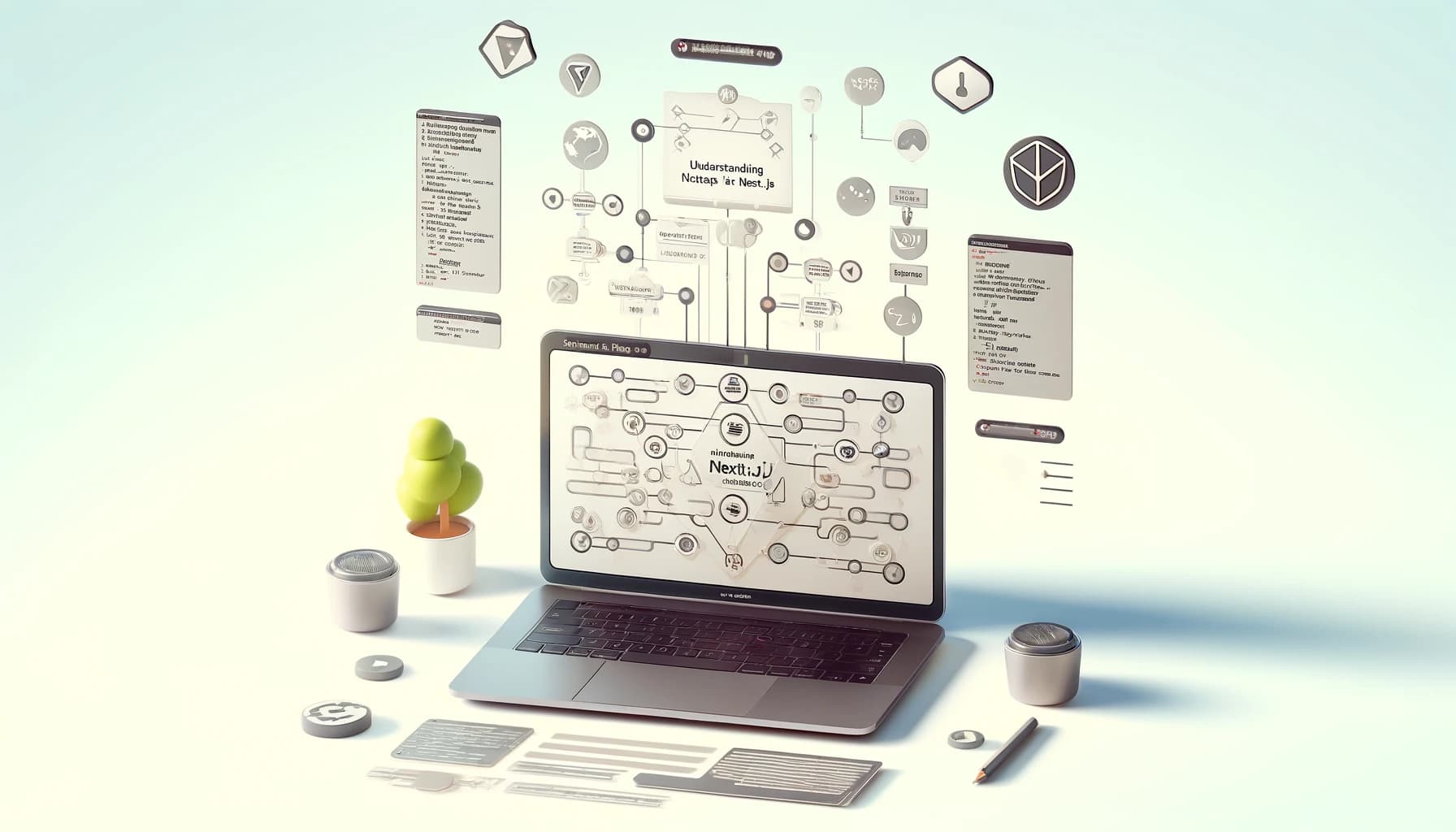
Outstatic Sitemap
Written on by Markus Ahlstrand.
I noticed that the default blog template in Outstatic
didn't generate any sitemap. Fortunately it's really simple to add this in nextjs as the Next.js App Router has built in support for generating sitemaps. You can use the sitemap.(js|ts)
file convention to programmatically generate a sitemap by exporting a default function that returns an array of URLs. If using TypeScript, a Sitemap
type is available.
import { MetadataRoute } from "next";
import { NEXT_PUBLIC_APP_URL } from "../lib/constants";
import { load } from "outstatic/server";
export default async function sitemap(): Promise<MetadataRoute.Sitemap> {
const db = await load();
const items = await db
.find(
{
collection: "posts",
status: "published",
},
["slug", "publishedAt"],
)
.toArray();
return items.map((item) => ({
url: `${NEXT_PUBLIC_APP_URL}/${item.slug}`,
lastModified: item.publishedAt,
}));
}
For this blog it would generate this xml file during the next build and make it available at https://ahlstrand.es/sitemap.xml
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
<url>
<loc>http://localhost:3000/rest-api-guidelines</loc>
<lastmod>2024-06-06T06:22:28.823Z</lastmod>
</url>
<url>
<loc>http://localhost:3000/oauth2-endpoints-parameters</loc>
<lastmod>2024-06-07T09:20:42.889Z</lastmod>
</url>
<url>
<loc>http://localhost:3000/outstatic-cms</loc>
<lastmod>2024-05-25T11:57:53.368Z</lastmod>
</url>
<url>
<loc>http://localhost:3000/outstatic-and-remark</loc>
<lastmod>2024-06-07T20:03:55.203Z</lastmod>
</url>
</urlset>
This might also be a good point to generate the indexes for flexsearch?