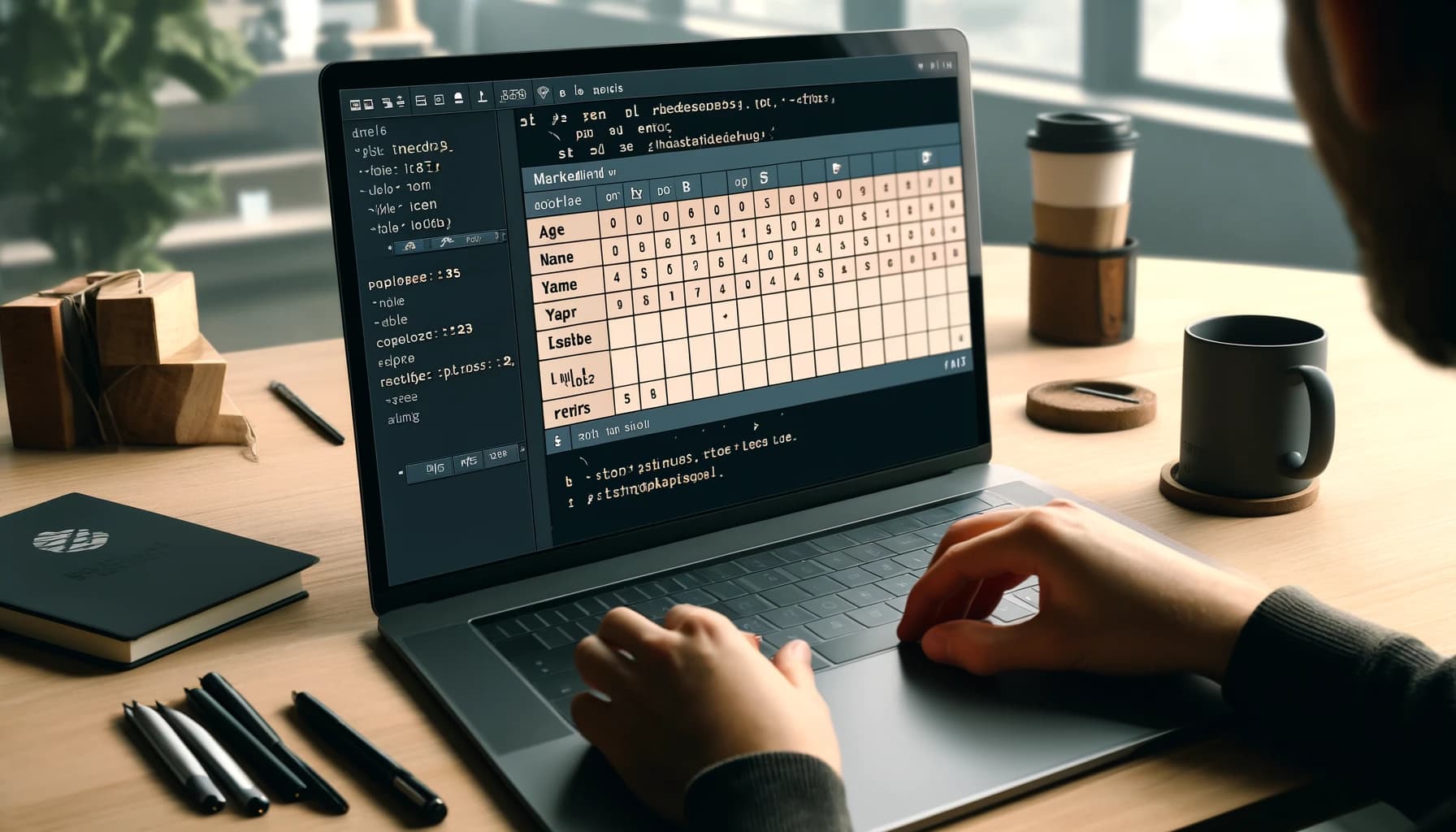
Outstatic and Remark/Rehype
After using Outstatic for while the only thing that didn't work straight out of the box was rendering the markdown tables that was created by the tiptap editor. I realized that the markdown tables actually aren't a part of the markdown core features but added in google's flavour of markdown. Outstatic is using the remark library for rendering the markdown as html and it turns out there's a remark-gfm plugin that adds support for tables, task lists, strikethroughs and a few other things.
The rendering is handled directly in the website project and not part of the Outstatic package, so it's easy to add. There's a markdownToHtml-helper that is used in all the content types:
import { remark } from "remark";
import html from "remark-html";
import remarkGfm from "remark-gfm";
export default async function markdownToHtml(markdown: string) {
const result = await remark().use(remarkGfm).use(html).process(markdown);
return result.toString();
}
Code Highlighting
When starting to add code snippets to the blog I also noticed that there's no highlighting of code which makes it look less fabulous. Getting this was slightly more involved as it doesn't seem to be a plug-and-play solution for this inside the remark ecosystem. Instead the most common solution seems to be to use rehype and specifically the rehype-pretty-code plugin. It was fairly easy to get it working, the only gotcha is that you need to use the html function from rehype instead of the one from remark.
import { remark } from "remark";
import remarkGfm from "remark-gfm";
import remarkRehype from "remark-rehype";
import rehypePrettyCode from "rehype-pretty-code";
import html from "rehype-stringify";
export default async function markdownToHtml(markdown: string) {
const result = await remark()
.use(remarkGfm)
.use(remarkRehype)
.use(rehypePrettyCode, {
theme: "nord",
keepBackground: true,
})
.use(html)
.process(markdown);
return result.toString();
}
And.. voila.. Now we have code snippets with code highlighting!